· development · 3 min read
Salesforce Apex Date Class Guide
The Date class provides methods to create and manipulate dates. It allows you to perform operations such as adding or subtracting days, obtaining the day of the week and formatting dates as strings.
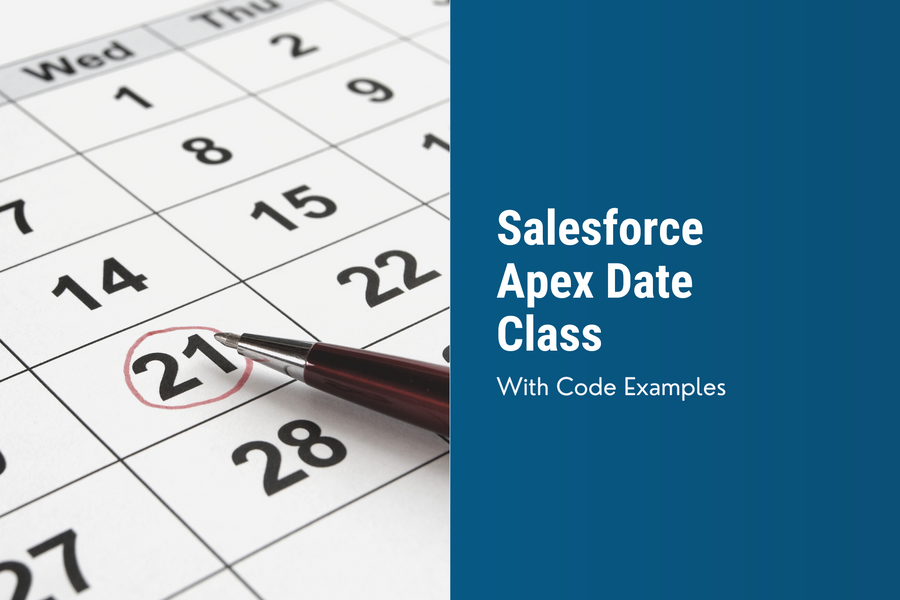
The Date class provides methods to create, manipulate, and compare dates. It allows you to perform operations such as adding or subtracting days, obtaining the day of the week, comparing dates for equality or ordering, and formatting dates as strings.
Introduction
The Date class in Apex provides methods to create, manipulate, and format dates.
Some of the common operations that can be performed using the Apex Date class include:
- Creating Date instances: You can create a Date object by specifying the year, month, and day using the Date.newInstance(year, month, day) method.
- Extracting components: The Date class allows you to extract individual components of a date such as year, month, and day using methods like year(), month(), and day().
- Manipulating dates: Apex provides methods to perform various operations on dates, such as adding or subtracting days using addDays() method, finding the difference between two dates using daysBetween(), and more.
- Formatting dates: The Date class includes methods like format() to convert a Date object into a formatted String representation based on the specified format or the user’s locale.
Apex Date Methods
Let’s take a look at the Apex Date methods.
addDays
The addDays method lets you add days and subtract days of a date.
The subtraction can be done by providing negative numbers.
Date addDays(Integer additionalDays))
- additionalDays (Integer): The number of days to add to the date.
- Date: The new date increased by the given number of days.
Date today = Date.today();
Date tomorrow = today.addDays(1);
addMonths
The addMonths method lets you add months and subtract months of a date.
The subtraction can be done by providing negative numbers.
Date addMonths(Integer additionalMonths))
- additionalMonths (Integer): The number of months to add to the date.
- Date: The new date increased by the given number of months.
Date today = Date.today();
Date nextMonth = today.addMonths(1);
addYears
The addYears method lets you add years and subtract years of a date.
The subtraction can be done by providing negative numbers.
Date addYears(Integer additionalYears))
- additionalYears (Integer): The number of years to add to the date.
- Date: The new date increased by the given number of years.
Date today = Date.today();
Date inTenYears = today.addYears(10);
day
To just get the day in the month of a date as a number, use the day method .
Integer day())
- Integer: The day of the month from this Date.
Date xmas = Date.newInstance(2023,12,24);
System.assertEquals(24,xmas.day());
dayOfYear
To just get the day in the year of a date as a number, use the dayOfYear method .
Integer dayOfYear())
- Integer: The day of the year from this Date.
Date xmas = Date.newInstance(2023,12,24);
System.assertEquals(358,xmas.dayOfYear());
daysBetween
Need to find out how much days are between two dates? Use the daysBetween method.
Integer daysBetween(Date secondDate))
- Integer: The number of days between the Date that invoked the method and the specified date.
Date xmas = Date.newInstance(2023,12,24);
Date sylvester = Date.newInstance(2023,12,31);
System.assertEquals(7,xmas.daysBetween(sylvester));
daysInMonth
To get the number of days in a month in a concrete year and month use the daysInMonth method.
static Integer daysInMonth(Integer year, Integer month))
- year (Integer): The year for which the number of days in month are requested.
- month (Integer): The month for which the number of days in month are requested. (where 1 represents January, 2 February ..)
- Integer: The number of days for the given month in the given year.
System.assertEquals(31,Date.daysInMonth(2023,1));
format
To get the date as a string in the format of your locale, you can simply call the format method.
String format())
- Date: The Date in a string representation using the locale of the current user context.
System.assertEquals('12/24/2023',xmas.format());
isLeapYear
Provide a year and find out, if it is a leap year or not.
static Boolean isLeapYear(Integer year))
- year (Integer): The year for that could be a leap year or not.
- Boolean: True, if the given date is a leap year, fasle if not.
System.assert(Date.isLeapYear(2024));
System.assertEquals(false,Date.isLeapYear(2025));
isSameDay
Are two dates at the same day? You can find out by using the isSameDay method.
Boolean isSameDay(Date dateToCompare))
- dateToCompare (Date): The date that might be on the same day as this date.
- Boolean: True, if this date is on the same day s the date to compare, fasle if not.
Date xmas = Date.newInstance(2023,12,24);
Date xmas2 = Date.newInstance(2023,12,24);
Date sylvester = Date.newInstance(2023,12,31);
System.assert(xmas.isSameDay(xmas2));
System.assertEquals(false,xmas.isSameDay(sylvester));
month
To just get the month of a date as a number, use the month method .
Integer month())
- Boolean: Month of the date. (where 1 represents January, 2 February ..)
Date xmas = Date.newInstance(2023,12,24);
System.assertEquals(12,xmas.month());
monthsBetween
Need to find out how much month are between two dates? Use the monthsBetween method.
Integer monthsBetween(Date secondDate))
- Integer: The number of months between the Date that invoked the method and the specified date.
Date xmas = Date.newInstance(2023,12,24);
Date sylvester = Date.newInstance(2024,12,31);
System.assertEquals(12,xmas.monthsBetween(sylvester));
newInstance
Create a date by providing year, month and day as number.
static Date newInstance(Integer year, Integer month, Integer day))
- Date: The date for of the given year, month and day.
Date xmas = Date.newInstance(2023,12,24);
System.assertEquals('12/24/2023',xmas.format());
parse
Convert a string to a date.
static Date parse(String stringDate))
- stringDate (String): The string that should be parsed to a date.
- Date: The result of parsing the string to date. (Working string formats depend on your local.)
Date.parse('12/24/2023');
today
Get the date of today.
static Date today())
- Date: Today's date.
Date.today();
toStartOfMonth
Get the first day of the month.
Date toStartOfMonth())
- Date: A date for the first day of the month of this date.
Date xmas = Date.newInstance(2023,12,24);
Date beginOfMonth = Date.newInstance(2023,12,1);
System.assertEquals(beginOfMonth,xmas.toStartOfMonth());
toStartOfWeek
Get the first day of the week.
Date toStartOfWeek())
- Date: The starting day of the week for the Date that invoked the method, based on the locale of the current user context.
Date xmas = Date.newInstance(2023,12,24);
Date beginOfWeek = Date.newInstance(2023,12,18);
System.assertEquals(beginOfWeek,xmas.toStartOfWeek());
valueOf
Get the date for a string.
static Date valueOf(String stringDate))
- stringDate (String): The string that should be used to create a date.
- Date: The result of converting the string to date.
Date xmas = Date.newInstance(2023,12,24);
System.assertEquals(xmas,Date.valueOf('2023-12-24'));
year
To just get the year of a date as a number, use the year method .
Integer year())
- Boolean: Year of the date.
Date xmas = Date.newInstance(2023,12,24);
System.assertEquals(2023,xmas.year());