· development · 3 min read
Salesforce Apex Map Class Guide
Learn about the Salesforce Apex Map Class, which is a collection type that stores data as key-value pairs. We will explore Apex maps by looking at many code examples.
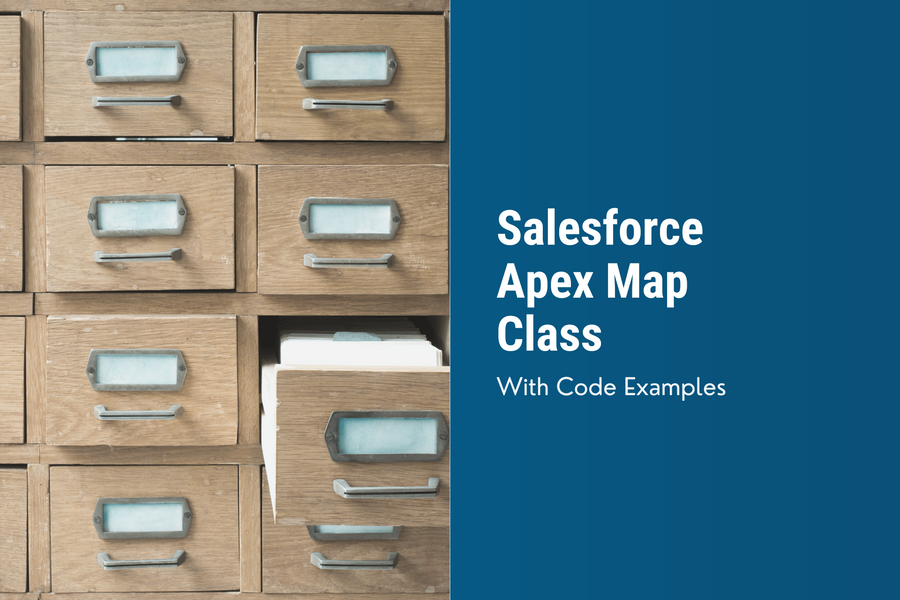
The Salesforce Apex Map class is a collection type that stores data as key-value pairs. It allows you to store and retrieve data based on a unique key that you define. The Map class is similar to a dictionary or a hash table in other programming languages.
Introduction
You will find detailed informations of the common map methods in this article.
But let’s get you started quickly.
We create a map.
Map<String, Integer> nameAndAge = new Map<String, Integer>();
The map is empty.
System.assert(nameAndAge.isEmpty());
We need to put something into the map.
nameAndAge.put('Sally', 33);
Now the map is not empty anymore. It has one key-value pair.
System.assertEquals(1,nameAndAge.size());
But how do we get the age of Sally out of the map?
System.assertEquals(33,nameAndAge.get('Sally'));
Finally, we clean up and remove Sally from the map.
nameAndAge.remove('Sally');
You’ve seen the basic interaction with a map!
Apex map methods
Let’s take a look at the most common Salesforce Apex Map methods.
clear
In case you want an empty map as if it was new, call the map clear method which removes all key-value pairs of the map.
Void clear())
- Void: The clear method returns nothing.
Map<String, Integer> nameAndAge = new Map<String, Integer>();
nameAndAge.put('Sally',37);
nameAndAge.put('John',21);
System.assertEquals(2,nameAndAge.size());
nameAndAge.clear();
System.assertEquals(0,nameAndAge.size());
containsKey
To check whether a key is already in a map or not, use the containsKey method.
Boolean containsKey(Object key))
- key (Object): The key to search for in the map.
- Boolean: True, if this map contains the given key. False, if the key is not in the map.
Map<String, Integer> nameAndAge = new Map<String, Integer>();
nameAndAge.put('Sally',37);
System.assertEquals(true,nameAndAge.containsKey('Sally'));
System.assertEquals(false,nameAndAge.containsKey('Mike'));
get
To retrieve a value from your map, use the get method and pass the required key as parameter.
Object get(Object key))
- key (Object): The key for the requested value.
- Object: The value for the given key. Null, if there is no value.
Map<String, Integer> nameAndAge = new Map<String, Integer>();
nameAndAge.put('Sally',37);
System.assertEquals(37,nameAndAge.get('Sally'));
System.assertEquals(null,nameAndAge.get('Mike'));
isEmpty
To check if a map is empty in Apex, use the isEmpty method.
Boolean isEmpty())
- Boolean: True, if there are no key-value pairs in the map, false if there are pairs int the map.
Map<String, Integer> nameAndAge = new Map<String, Integer>();
System.assertEquals(true, nameAndAge.isEmpty());
nameAndAge.put('Sally',37);
System.assertEquals(false, nameAndAge.isEmpty());
keySet
Need to know, which keys are used within your map? Use the keySet method to retrieve all keys of a map.
Set<Object> keySet())
- Set<Object>: A set that includes all the keys present in the map.
Map<String, Integer> nameAndAge = new Map<String, Integer>();
nameAndAge.put('Sally',37);
nameAndAge.put('Mike',21);
Set<String> keys= nameAndAge.keySet();
System.assert(keys.contains('Sally'));
System.assert(keys.contains('Mike'));
put
The map put method lets you add key-value pairs to a map.
Object put(Object key, Object value))
- key (Object): The key which is used to find the value.
- value (Object): The value that can be found under the given key.
- Object: If the map previously contained a mapping for this key, the old value is returned. If there is no old value, null is returned.
Map<String, Integer> myMap = new Map<String, Integer>();
Object oldValue = myMap.put('Sally', 33);
System.assertEquals(null,oldValue);
oldValue = myMap.put('Sally', 27);
System.assertEquals(33,oldValue);
System.assertEquals(27,myMap.get('Sally'));
remove
The map remove method lets you remove a key-value pair of your map.
Object remove(Object key))
- key (Object): The string that should be replaced.
- Object: The value of the removed object key-value pair. Null, if there is no value for the given key.
Map<String, Integer> myMap = new Map<String, Integer>();
Object value = myMap.remove('Sally');
System.assertEquals(null,value);
myMap.put('Sally', 33);
value = myMap.remove('Sally');
System.assertEquals(33,value);
size
The size method lets you check how many key-value pairs are in you map-
Integer size())
- Integer: The count of key-value pairs present in the map.
Map<String, Integer> myMap = new Map<String, Integer>();
System.assertEquals(0,myMap.size());
myMap.put('Sally', 33);
System.assertEquals(1,myMap.size());
values
To get all values from a map, use the Apex map values method.
List<Object> values())
- List<Object>: A list that includes all the values stored in the map.
Map<String, Integer> myMap = new Map<String, Integer>();
myMap.put('Sally', 33);
myMap.put('Mike', 35);
List<Integer> values = myMap.values();
System.assertEquals(true,values.contains(33));
System.assertEquals(true,values.contains(35));