· development · 3 min read
Salesforce Apex Set Class Guide
The Apex Set class provides various methods to work with sets like adding elements, removing elements, accessing elements and searching elements.
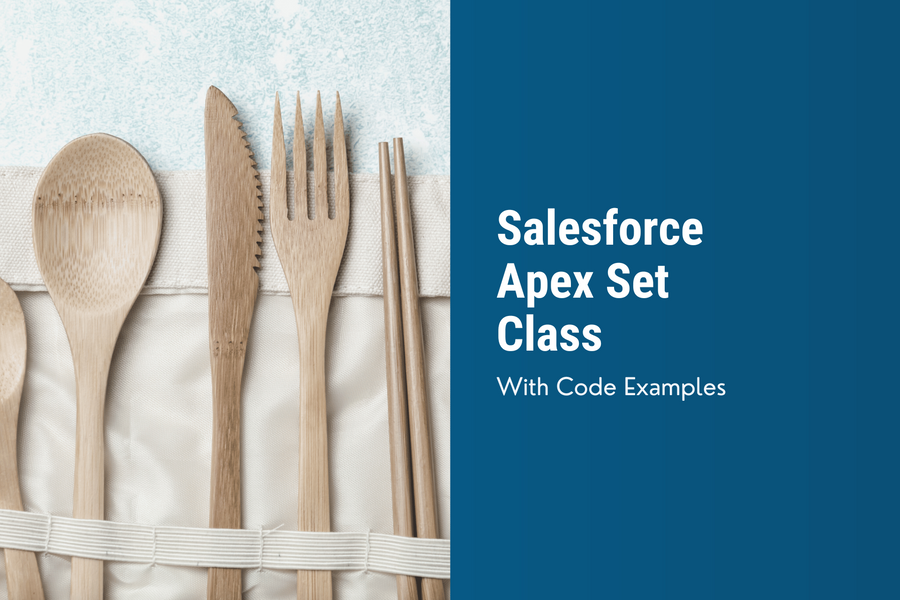
Introduction
In Salesforce Apex, a set is a collection that allows you to store unique elements of the same data type. A set is similar to a mathematical set, where each element is unique and there is no specific order maintained. In Salesforce Apex, the Set class provides methods and properties to work with sets efficiently.
Here are some key features and characteristics of Salesforce Apex sets:
- Unique Elements: Sets in Apex only store unique elements. If you attempt to add a duplicate element to a set, it will be ignored.
- Unordered Collection: Elements in a set do not have a specific order or index position. The order of elements is not guaranteed and can change.
- Homogeneous Elements: A set in Apex can store elements of the same data type. For example, a set can hold a collection of strings, integers, custom objects, or any other data type.
- Dynamic Size: Sets in Apex are dynamic, meaning they can grow or shrink as elements are added or removed. You don’t need to specify the initial size of the set.
- Methods and Operations: The Apex Set class provides various methods and operations to manipulate and work with sets. These include adding elements, removing elements, checking for element presence and difference, and more.
Salesforce Apex sets are commonly used to store and manage collections of unique data, such as unique records or unique values from a larger dataset. They are particularly useful when you want to ensure uniqueness or perform set operations on a collection of data.
Apex set methods
Let’s take a look at some methods of the Set class.
add
To add an element to a set, use the add method.
Note: since sets only contain unique elements, add does nothing if the element is already in the set.
Boolean add(Object setElement))
- setElement (Object): The element that should be added to the set.
- Boolean: Returns true, if the set was changed by adding an element. False, if the element was already in the set.
Set<String> fruits = new Set<String>();
Boolean setUpdated = fruits.add('apple');
System.assertEquals(true,setUpdated);
addAll
To add a list of elements to a set, use the addAll method. All items from the list, that are not already in the set will be added.
Boolean addAll(List<Object> fromList))
- fromList (List<Object>): A list of elements that should be added to this set.
- Boolean: Returns true, if the set was changed by adding at least one element. False, if none of the elements was added to the set, because they were already in the set.
Set<String> fruits = new Set<String>();
Boolean setUpdated = fruits.add('apple');
List<String> moreFruits = new List<String>();
moreFruits.add('banana');
moreFruits.add('orange');
fruits.addAll(moreFruits);
System.assertEquals(3,fruits.size());
List<String> evenMoreFruits = new List<String>();
evenMoreFruits.add('banana');
fruits.addAll(evenMoreFruits);
System.assertEquals(3,fruits.size());
The addAll method also takes other sets as input. All items of the added set that are not already in the original set will be added.
Boolean addAll(Set<Object> fromSet))
- fromSet (Set<Object>): A set of elements that should be added to this set.
- Boolean: Returns true, if the set was changed by adding at least one element. False, if none of the elements was added to the set, because they were already in the set.
Set<String> fruits = new Set<String>();
Boolean setUpdated = fruits.add('apple');
Set<String> moreFruits = new Set<String>();
moreFruits.add('banana');
moreFruits.add('orange');
fruits.addAll(moreFruits);
System.assertEquals(3,fruits.size());
Set<String> evenMoreFruits = new Set<String>();
evenMoreFruits.add('banana');
fruits.addAll(evenMoreFruits);
System.assertEquals(3,fruits.size());
clear
To remove all elements of a set, use the clear method.
Void clear())
- Void: The clear method returns nothing.
Set<String> fruits = new Set<String>();
fruits.add('apple');
System.assertEquals(1,fruits.size());
fruits.clear();
System.assertEquals(0,fruits.size());
contains
To find out, whether an element is in a set or not, use the contains method.
Boolean contains(Object setElement))
- setElement (Object): The element that might be within in the set.
- Boolean: True, if the set includes the specified element.
Set<String> fruits = new Set<String>();
fruits.add('apple');
System.assertEquals(1,fruits.size());
fruits.clear();
System.assertEquals(0,fruits.size());
containsAll
To verify that as list is a subset of a set you can use the containsAll method. ContainsAll returns true if all aof the elements in the list are in the set.
Boolean containsAll(List<Object> listToCompare))
- listToCompare (List<Object>): List which elements might be in the set.
- Boolean: True, if the set contains all the elements in the specified list.
Set<String> fruits = new Set<String>();
fruits.add('apple');
fruits.add('banana');
fruits.add('orange');
List<String> moreFruits = new List<String>();
moreFruits.add('apple');
moreFruits.add('banana');
System.assertEquals(true,fruits.containsAll(moreFruits));
moreFruits.add('melon');
System.assertEquals(false,fruits.containsAll(moreFruits));
isEmpty
To check whether a set is empty or nut, use the isEmpty method.
Boolean isEmpty())
- Boolean: True, if the set contains no elements."
Set<String> fruits = new Set<String>();
System.assertEquals(true,fruits.isEmpty());
fruits.add('apple');
System.assertEquals(false,fruits.isEmpty());
remove
To remove a single item from a set, use the remove method.
Boolean remove(Object setElement))
- setElement (Object): The element that should be removed from the set.
- Boolean: True, if the call resulted in a change to the original set.
Set<String> fruits = new Set<String>();
fruits.add('apple');
System.assertEquals(true,fruits.contains('apple'));
fruits.remove('apple');
System.assertEquals(false,fruits.contains('apple'));
removeAll
To remove multiple items of a set in one call, use the removeAll method.
There two variants of the removeAll method. One takes a list as input and one a set.
This is removeAll with a list.
Boolean removeAll(List<Object> listOfElementsToRemove))
- listOfElementsToRemove (List<Object>): The list of elements that should be removed from the set.
- Boolean: True, if the set was changed by removing elements.
List<String> moreFruits = new List<String>();
moreFruits.add('apple');
moreFruits.add('melon');
moreFruits.add('orange');
fruits.removeAll(moreFruits);
System.assertEquals(1,fruits.size());
System.assertEquals(true,fruits.contains('banana'));
This is removeAll with a set.
Boolean removeAll(Set<Object> setOfElementsToRemove))
- setOfElementsToRemove (Set<Object>): The set of elements that should be removed from the set.
- Boolean: True, if the set was changed by removing elements.
Set<String> fruits = new Set<String>();
fruits.add('apple');
fruits.add('banana');
fruits.add('orange');
Set<String> moreFruits = new Set<String>();
moreFruits.add('apple');
moreFruits.add('melon');
moreFruits.add('orange');
fruits.removeAll(moreFruits);
System.assertEquals(1,fruits.size());
System.assertEquals(true,fruits.contains('banana'));
retainAll
You need to know the intersection between a set and a list or between two sets? Use the retainAll method.
RetainAll removes all elements that are not present in both sets or in the set and the list.
Here is the retainAll method with a list.
Boolean retainAll(List<Object> listOfElementsToRetain))
- listOfElementsToRetain (List<Object>): The list of elements to retain.
- Boolean: If the method caused a change to the original set, it will return true.
Set<String> fruits = new Set<String>();
fruits.add('apple');
fruits.add('banana');
fruits.add('orange');
List<String> moreFruits = new List<String>();
moreFruits.add('apple');
moreFruits.add('melon');
moreFruits.add('orange');
fruits.retainAll(moreFruits);
System.assertEquals(2,fruits.size());
System.assertEquals(true,fruits.contains('apple'));
System.assertEquals(true,fruits.contains('orange'));
Here is the retainAll method with a set.
Boolean retainAll(Set<Object> setOfElementsToRetain))
- setOfElementsToRetain (Set<Object>): The set of elements to retain.
- Boolean: If the call resulted in a change to the original set, this method returns true.
Set<String> fruits = new Set<String>();
fruits.add('apple');
fruits.add('banana');
fruits.add('orange');
Set<String> moreFruits = new Set<String>();
moreFruits.add('apple');
moreFruits.add('melon');
moreFruits.add('orange');
fruits.retainAll(moreFruits);
System.assertEquals(2,fruits.size());
System.assertEquals(true,fruits.contains('apple'));
System.assertEquals(true,fruits.contains('orange'));
size
To get the number of elements within a set, use the size method.
Integer size())
- Integer: The count of elements in the set.
Set<String> fruits = new Set<String>();
System.assertEquals(0,fruits.size());
fruits.add('apple');
System.assertEquals(1,fruits.size());