· development · 2 min read
Salesforce Apex Switch Case Explained
A guide with many code examples on how to use switch case in Apex.
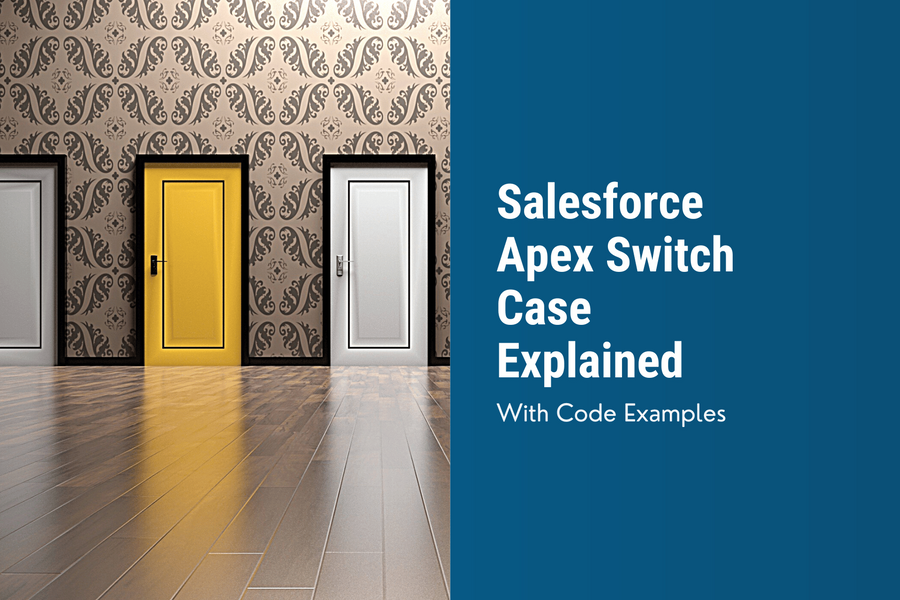
A switch statement is a control flow structure in programming that allows the execution of different blocks of code depending on the value of a given expression or variable.
Switch statements are commonly used when there are multiple conditions to be checked, and each condition may lead to a different set of actions. Instead of writing multiple if statements, a switch statement provides a more concise and readable way to handle multiple cases.
Apex Switch Case
If statements that compare a variable against multiple values are hard to read. Switch statements provide a cleaner way to express the same.
In Apex Switch statements can be used with the following types:
- Enum
- Integer
- Long
- sObject
- String
Below you see an switch case string example.
Apex Switch Case Examples
Let’s take a look at an Apex switch statement example:
String fruit = 'apple';
switch on fruit {
when 'apple' {
System.debug('You chose an apple.');
}
when 'banana' {
System.debug('You chose a banana.');
}
when 'orange' {
System.debug('You chose an orange.');
}
when else {
System.debug('You chose a fruit that is not in our list.');
}
}
This basic switch case in Apex example will print “You chose an apple.” to the debug log.
Switch Statement Default
The following example demonstrates a switch case with an enum. As you see, there is no when clause for TRIAL and HOBBY. They are both handled by the default clause in “when else”.
Note: The “when else” clause needs to be the last option in the switch statement.
public enum Plan {TRIAL, HOBBY, PROFESSIONAL, ENTERPRISE}
Plan selectedPlan = Plan.HOBBY;
switch on selectedPlan {
when PROFESSIONAL {
System.debug('$10');
}
when ENTERPRISE {
System.debug('$250');
}
when else {
System.debug('It's free.');
}
}
Switch Case Multiple Values
You can provide multiple values to a the when clause of a switch case.
Take a look at the switch case example below. Banana and lemon are both yellow. You can simply name multiple options by separate them by commas.
String fruit = 'banana';
switch on fruit {
when 'banana','lemon' {
System.debug('yellow');
}
when 'strawberry' {
System.debug('red');
}
when else {
System.debug('unknown');
}
}