· development · 2 min read
Salesforce Apex Try Catch Finally Explained
Learn how to do error handling in Apex with try catch blocks and understand the finally clause by many try catch examples.
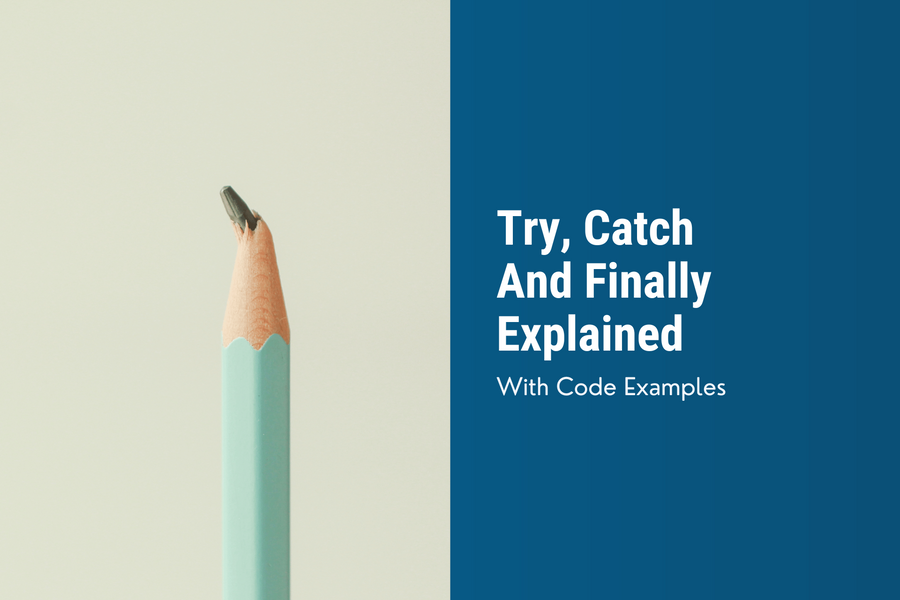
How try catch finally works
In Apex, the try-catch-finally block is used for exception handling.
It allows you to handle exceptions that may occur during the execution of your code and provides a way to perform necessary cleanup actions.
Let’s take a look at each block.
Try Block
The code that might throw an exception is placed within the try block.
If an exception occurs within this block, it is immediately caught, and the execution jumps to the catch block.
try{
doSomethingDangerous();
}catch (Exception ex){
// clean up
}
Catch Block
The catch block is used to handle the caught exception.
try{
Integer result = 10 / 0; // throws MathException, because division by zero is not allowed.
}catch (System.MathException ex){
// set result to -1 in case of an error
result = -1;
}
It specifies the type of exception that can be caught, allowing you to handle specific types of exceptions differently.
See the example for different exception types that might be thrown:
- StringException: Because an empty string has no first character
- MathException: Because division by zero is not allowed
Integer myNumber = 21;
String myString = '';
try {
myNumber = myNumber / 0;
myString = myString.substring(0, 1); // will not be executed, because of the error before
} catch (System.StringException ex) {
System.debug('Catching StringException'); // NOT executed
} catch (System.MathException ex2) {
System.debug('Catching MathException'); // Executed!
}
// => DEBUG Output: Catching MathException
Note that if an exception occurs and is caught, the code after the catch block is not executed.
So can have multiple catch blocks for different exception types or a single catch block to handle multiple exception types.
In the following example all exception are caught by the catch clause, because all other exceptions inherit from exception.
Integer myNumber = 21;
String myString = '';
try {
myNumber = myNumber / 0;
myString = myString.substring(0, 1);
} catch (Exception ex) {
System.debug('I catch every exception, because all other exceptions inherit from exception.');
}
Finally Block
The finally block is optional and follows the catch block.
The code within the finally block is executed regardless of whether an exception occurred or not.
try {
// may throw exception or not
} catch (Exception ex) {
// handle exception
} finally {
System.debug('I get ALWAYS executed!');
}
It is commonly used to perform cleanup actions, such as closing open connections or releasing resources, ensuring they are always executed.
Although it is not very common, there can be try finally without catch.
This way you can clean up resources, even if you return early in a method.
public class MyClass {
void foo() {
try {
if (1 > 0) {
return;
}
System.debug('I will not be executed.');
} finally {
System.debug('Goodbye.'); // I will be executed!
}
}
}
new MyClass().foo();