· development · 10 min read
Salesforce Apex String Class Guide
Learn about the Salesforce Apex String Class, which is a key data type in Apex used to represent text values. We will explore Apex strings by looking at examples on manipulating, comparing and converting strings.
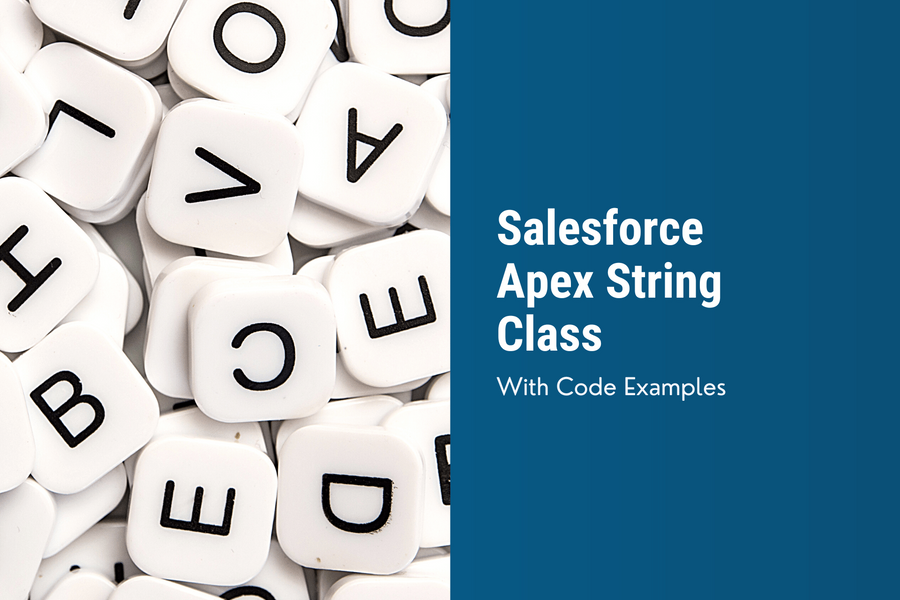
The approach of this article is to provide solutions to common tasks/problems concerning Apex Strings. Click on the link, in case you are looking for the Apex Reference Guide of the String Class.
Do not forget to bookmark this article for later reference.
Salesforce Apex String Methods
Before we dive into the solutions for common tasks with strings, let’s get to know some common Salesforce Apex String methods.
String methods Salesforce:
Split
Need to convert a String to a list or array. The split function is there for you.
String fruits = ' apple , banana , orange ';
String[] result = fruits.trim().split('\s*,\s*');
System.debug(result);
// => ('apple','banana','orange')
Follow the link for more about the Salesforce Apex Split method.
Contains
The contains method lets you check if string contains a substring.
String text = 'I am John.';
Boolean result = text.contains('John');
System.assertEquals(true, result);
Be aware, the contains method is case-sensitive.
String text = 'I am John.';
Boolean result = text.contains('john');
System.assertEquals(false, result);
Follow the link for more about the Salesforce Apex Contains method.
Replace
Apex offers two methods to replace strings within a string. The most common is the replace method. Just pass in which string should be replaced by what string.
String result = '123-456-789'.replace('-','+');
System.assertEquals('123+456+789',result);
Follow the link for more about the Salesforce Apex Replace method.
String replace all
A more advanced replace scenario is using replaceAll. In the example we are replacing all numbers with plus signs.
String result = '123-456-789'.replaceAll('[\d]','+');
System.assertEquals('+++-+++-+++',result);
ValueOf
The valueOf methods of different types are used to do type conversions.
Here is an example of how to convert a number to a string.
Integer aNumber = 123;
String result = String.valueOf(aNumber);
System.assertEquals('123', result);
You find more examples with other types in the conversion chapter of this article.
StartsWith
This is how to check if a Salesforce Apex string starts with a certain string.
String fruit = 'apple';
System.assertEquals(true, fruit.startsWith('a'));
System.assertEquals(false, fruit.startsWith('A'));
Note: The startsWith method is case-sensitive.
If you want to do a case-insensitive check if a string begins with a another string, use the startsWithIgnoreCase method.
System.assertEquals(true, 'apple'.startsWithIgnoreCase('A'));
EndsWith
This is how to check if a Salesforce Apex string ends with a certain string.
String fruit = 'apple';
System.assertEquals(true, fruit.endsWith('e'));
System.assertEquals(false, fruit.endsWith('E'));
Note: The endsWith method is case-sensitive.
If you want to do a case-insensitive check if a string end with a another string, use the endsWithIgnoreCase method.
System.assertEquals(true, 'apple'.endsWithIgnoreCase('E'));
String manipulation
Here you find lots of examples for modifying strings, like concating, joining, splitting, appending and replacing strings.
String concatenation / Append String
To concat strings with Apex, you can use the plus operator. See how to concatenate strings with Apex in the example:
String result = 'Sales' + 'force';
System.debug(result); // => Salesforce
System.assertEquals('Salesforce',result);'
This is how to append string in Apex.
String Escape Sequences
To escape a certain character you can use the backslash like shown in the following example:
Escape single quotes
Place a backslash in front of the single quote to escape it. See code example for escaping single quotes:
String result = 'Joe\'s diner';
System.debug(result);
// => Joe's diner
This is how to add single quotes in an Apex string.
Other common escape sequences
Take a look at the following list, to see how to escape special characters in Apex.
- \\ Backslash
- \’ Single quote
- \” Double quote
- \n New line
- \r Carriage return
- \t Tab
Remove special characters from string
Sometimes you need to get rid of all special characters. To remove special characters from a string, you can use the replaceAll method to replace all occurrences of special charters by nothing.
Take a look at the example:
String text = '({F]%*o:,r-#)ce!^H.}]o[@$w?';
text = text.replaceAll('[^a-zA-Z0-9\s+]', '');
System.assertEquals('ForceHow', text);
This is how to remove special characters from a string in Apex.
Remove whitespaces
To remove all spaces from a string, there is the deleteWhitespace method.
System.assertEquals('abc', ' a b c '.deleteWhitespace());
String join
To join a list to a string, you can use the static join method like that.
List<String> abc = new List<String> {'a','b','c'};
String result = String.join(abc,',' );
System.assertEquals('a,b,c', result);
Get first character of string
String result = 'abc'.substring(0,1);
System.assertEquals('a', result);
Note: An empty string would cause an StringException, because length zero minus one would not work.
String emtpyString = '';
try {
emtpyString.substring(0,emtpyString.length()-1);
System.Assert.fail();
} catch(Exception e){
System.assertEquals('System.StringException',e.getTypeName());
}
In case you are not sure, that the string is not empty do isEmpty check upfront.
Remove last character from string
You can remove the last character of string by building a substring that ends before the last charater.
String fruits = 'apple,banana,orange,';
String result = fruits.substring(0,fruits.length()-1);
System.assertEquals('apple,banana,orange', result);
Be careful: You should check upfront, that the string is not empty. An empty string would cause a StringException.
Use removeEnd in case you know which charater should be removed.
String fruits = 'apple,banana,orange,';
String result = fruits.removeEnd(',');
System.assertEquals('apple,banana,orange', result);
Comparison
String null or empty
To assure that a given string is not null or empty use the isEmpty method.
System.assertEquals(true, String.isEmpty(null));
System.assertEquals(true, String.isEmpty(''));
// FALSE!
System.assertEquals(false, String.isEmpty('a'));
System.assertEquals(false, String.isEmpty(' '));
String isBlank vs isEmpty
Beside the isEmpty method there is also a isBlank method. But what is the difference?
There is only one difference: A string that only contains whitespaces is blank but not empty.
System.assertEquals(true, String.isBlank(''));
System.assertEquals(true, String.isBlank(' '));
System.assertEquals(true, String.isEmpty(''));
// FALSE!
System.assertEquals(false, String.isEmpty(' '));
Conversion
Learn how to convert a Salesforce Apex String to another type.
String to boolean
This is how you convert a Salesforce Apex String to a boolean.
String trueStr = 'true';
Boolean bool = Boolean.valueOf(trueStr);
System.assertEquals(true, bool);
String to number
This is how you convert a Salesforce Apex String to a number.
String numberStr = '123';
Integer result = Integer.valueOf(numberStr);
System.assertEquals(123, result);
This exampleshowed,how to convert string to decimal in Apex.
Number to string
This is how you convert a Salesforce Apex number to a string.
Example how to convert decimal to string:
Integer aNumber = 123;
String result = String.valueOf(aNumber);
System.assertEquals('123', result);
This is how to convert integer to string in Apex.
Date to string
To convert a date to a string use the static valueOf method of the String class.
Date myDate = Date.newinstance(2023, 12, 24);
String result = String.valueOf(myDate);
System.assertEquals('2023-12-24',result);
String to date
To convert a date to a string use the static valueOf method of the Date class.
String dateAsString = '2023-12-24';
Date result = Date.valueOf(dateAsString);
Date expected = Date.newinstance(2023, 12, 24);
System.assertEquals(expected,result);