· development · 2 min read
Salesforce Apex String Split Method Explained By Examples
Learn how to split a string by using the Apex String split method. We explain the split method and get into common use cases by showing you examples of how to split a string in Apex.
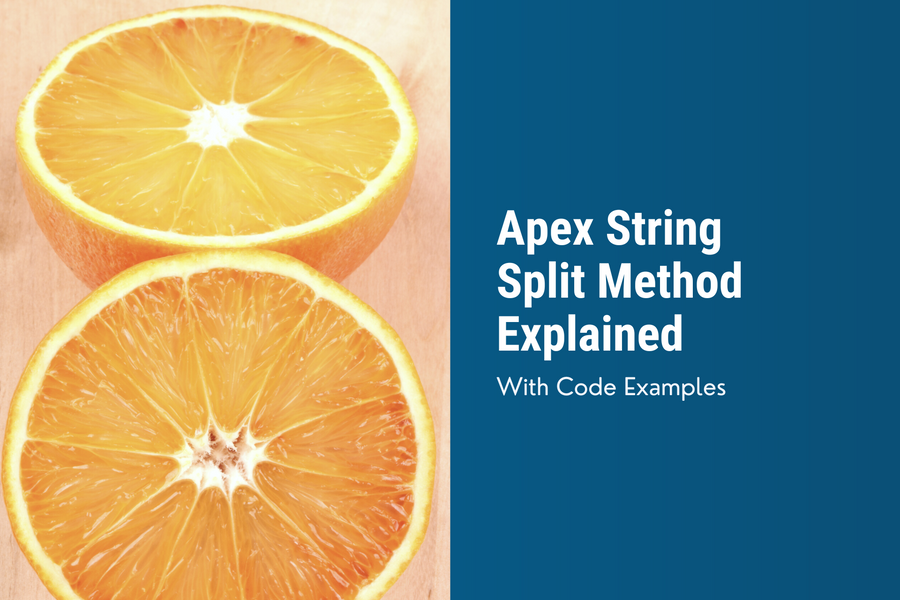
In my experience, the reason for most problems with the Salesforce Apex split method is that the parameter takes a regular expression. In some cases, this leads to unexpected results. Therefore we have a lot of examples that target the most common issues.
Apex String Split Method
Let’s start by taking a look at the details of the split method of the String class.
String[] split(String regEx))
- regEx (String): The regular expression that defines how to split this string.
- String []: A String array containing the substrings resulting from the given regular expression.
String str = 'abc-def-ghi';
str.split('-');
// => ['abc','def','ghi']
Now that we know, how the split method ca be used, let’s see more examples of the method in action.
Examples - Apex String split method
Split to list
This is how to convert a string to a list.
String fruits = 'apple,banana,orange';
List<String> result = fruits.split(',');
System.debug(result);
// => ('apple','banana','orange')
Split and trim
To split a string and get rid of any leading or trailing whitespaces, you can use the following regular expression in combination of a trim.
String fruits = ' apple , banana , orange ';
String[] result = fruits.trim().split('\s*,\s*');
System.debug(result);
// => ('apple','banana','orange')
Split by dot
To split a string by a dot might look trivial to you. But you have to be careful! The dot has a meaning within regular expressions, so you need to escape the dot by two backslashes.
String fruits = 'apple.banana.orange';
String[] result = fruits.split('\\.');
System.debug(result);
// => ('apple','banana','orange')
Split by character
Splitting a String by a character is easy. Just keep in mind, that the character is casesensitive.
String fruits = 'appleXbananaXorange';
String[] result = fruits.split('X');
System.debug(result);
// => ('apple','banana','orange')
Split a string by space
To split a string by space simply pass the whitespace to the split method.
String fruits = 'apple banana orange';
String[] result = fruits.split(' ');
System.debug(result);
// => ('apple','banana','orange')
Split by comma
To split a string by comma simply pass the comma to the split method.
String fruits = 'apple,banana,orange';
String[] result = fruits.split(',');
System.debug(result);
// => ('apple','banana','orange')
Split by new line
To split a string by a line break, you can use the ‘\n’ as an alias for the new line.
String fruits = 'apple\nbanana\norange';
String[] result = fruits.split('\n');
System.debug(result);
// => ('apple','banana','orange')
Split by pipe
To split a string by a pipe symbol might look trivial to you. But you have to be careful! The pipe has a meaning within regular expressions, so you need to escape the pipe symbol by two backslashes.
String fruits = 'apple|banana|orange';
String[] result = fruits.split('\|');
System.debug(result);
// => ('apple','banana','orange')